How to access airport information?
The traffic library provides an airport database with facilitated access to runway information and apron layout.
- class traffic.data.basic.airports.Airports(data=None)
An airport is accessible via its ICAO or IATA code. In case of doubt, use the search method.
The representation of an airport is based on its geographical footprint. Contours are fetched from OpenStreetMap (you need an Internet connection the first time you call it) and put in cache.
A database of major world airports is available as:
>>> from traffic.data import airports
Airports information can be accessed with attributes:
>>> airports["EHAM"].latlon (52.3086, 4.7639) >>> airports["EHAM"].iata 'AMS' >>> airports["EHAM"].name 'Amsterdam Airport Schiphol'
- __getitem__(name)
Any airport can be accessed by the bracket notation.
>>> from traffic.data import airports >>> airports["EHAM"] Airport(icao='EHAM', iata='AMS', name='Amsterdam Airport Schiphol', country='Netherlands', latitude=52.308601, longitude=4.76389, altitude=-11)
- extent(extent, buffer=0.5)
Selects the subset of data inside the given extent.
- Parameters:
extent (
str
|ShapelyMixin
|tuple
[float
,float
,float
,float
]) –The parameter extent may be passed as:
a string to query OSM Nominatim service;
the result of an OSM Nominatim query (
ShapelyMixin
);any kind of shape (
ShapelyMixin
, includingAirspace
);extent in the order: (west, east, south, north)
buffer (
float
) – As the extent of a given shape may be a little too strict to catch elements we may expect when we look into an area, the buffer parameter (by default, 0.5 degree) helps enlarging the area of interest.
- Return type:
Optional
[Self
]
This works with databases like
Airways
,Airports
orNavaids
.>>> from traffic.data import airways >>> airways.extent(eurofirs['LFBB']) route id navaid latitude longitude ━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ A25 6 GODAN 47.64 -1.96 A25 7 TMA28 47.61 -1.935 A25 8 NTS 47.16 -1.613 A25 9 TIRAV 46.6 -1.391 A25 10 LUSON 46.5 -1.351 A25 11 OLERO 45.97 -1.15 A25 12 MAREN 45.73 -1.062 A25 13 ROYAN 45.67 -1.037 A25 14 BMC 44.83 -0.7211 A25 15 SAU 44.68 -0.1529 ... (703 more lines)
>>> from traffic.data import airports >>> airports.extent("Bornholm") name country icao iata latitude longitude ━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ Bodilsker Airstrip Denmark DK-0027 nan 55.06 15.05 Bornholm Airport Denmark EKRN RNN 55.06 14.76 Ro Airport Denmark EKRR nan 55.21 14.88
>>> from traffic.data import navaids >>> navaids['ZUE'] Navaid('ZUE', type='NDB', latitude=30.9, longitude=20.06833333, altitude=0.0, description='ZUEITINA NDB', frequency='369.0kHz') >>> navaids.extent('Switzerland')['ZUE'] Navaid('ZUE', type='VOR', latitude=47.59216667, longitude=8.81766667, altitude=1730.0, description='ZURICH EAST VOR-DME', frequency='110.05MHz')
- search(name)
- Parameters:
name (
str
) – refers to the IATA or ICAO code, or part of the country name, city name of full name of the airport.- Return type:
>>> from traffic.data import airports >>> airports.query('type == "large_airport"').search('Tokyo') name country icao iata latitude longitude ━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ Narita International Airport Japan RJAA NRT 35.76 140.4 Tokyo Haneda International Airport Japan RJTT HND 35.55 139.8
Jupyter representation
Airports offer special display outputs in Jupyter Notebook based on their geographic footprint.
from traffic.data import airports
airports['EHAM']
Airport
Amsterdam Airport Schiphol (Netherlands)EHAM/AMS
(52.308601, 4.76389), altitude: -11
OpenStreetMap representation
The ._openstreetmap()
method queries information about the airport layout
from OpenStreetMap thanks to the cartes
library. This data is intended to be accessed through attributes corresponding
to aeroway
tags, e.g. apron
, taxiway
, parking_position
,
gate
, etc.
If you spot any error in data, consider contributing to OpenStreetMap.
airports["EHAM"].parking_position.head()
id_ | type_ | latitude | longitude | aeroway | ref | |
---|---|---|---|---|---|---|
105 | 2066153573 | node | 52.296220 | 4.745941 | parking_position | R81R |
106 | 2066153574 | node | 52.294546 | 4.741574 | parking_position | R72R+ |
107 | 2066153576 | node | 52.294924 | 4.742542 | parking_position | R74R |
108 | 2066153580 | node | 52.296600 | 4.746832 | parking_position | R82R |
109 | 2066153581 | node | 52.295340 | 4.743634 | parking_position | R77R |
airports["EHAM"].taxiway.head()
id_ | type_ | latitude | longitude | geometry | aeroway | ref | name | surface | width | |
---|---|---|---|---|---|---|---|---|---|---|
1039 | 8586291 | way | 52.315922 | 4.746750 | LINESTRING (4.74667 52.31486, 4.74663 52.31527... | taxiway | NaN | N5 | concrete | 23 |
1043 | 8586299 | way | 52.325770 | 4.740635 | LINESTRING (4.73934 52.32385, 4.73941 52.32417... | taxiway | NaN | W3 | concrete | 23 |
1044 | 8586300 | way | 52.320267 | 4.740608 | LINESTRING (4.74188 52.31959, 4.74185 52.31981... | taxiway | W5 | W5 | concrete | 23 |
1045 | 8586301 | way | 52.316439 | 4.740028 | LINESTRING (4.73884 52.31843, 4.73896 52.31796... | taxiway | W6 | W6 | concrete | 23 |
1046 | 8586302 | way | 52.311481 | 4.739513 | LINESTRING (4.73839 52.31354, 4.73844 52.31316... | taxiway | W7 | W7 | concrete | 23 |
Warning
The GeoDataFrame associated with the represented data is accessible through
the .data
attribute.
Altair representation
footprint
is True by default and shows the OpenStreetMap representation;runways
is True by default and represents the runways in bold lines.Default parameters arestrokeWidth=4, stroke="black"
which can be overridden if a dictionary is passed in place of the boolean;labels
is True by default and represents the runway numbers.Labels are automatically rotated along the runway bearing.Default parameters arebaseline="middle", dy=20, fontSize=18
which can be overridden if a dictionary is passed in place of the boolean;
import altair as alt
from traffic.data import airports
from traffic.data.samples import belevingsvlucht
chart = (
alt.layer(
airports["EHAM"].geoencode(
footprint=True, # True by default
runways=True, # default parameters
labels=dict(fontSize=12, fontWeight=400, dy=10), # font adjustments
),
belevingsvlucht.first(minutes=1)
.geoencode()
.mark_line(color="steelblue"),
belevingsvlucht.last(minutes=6)
.geoencode()
.mark_line(color="orangered"),
)
.properties(
width=500, height=500, title="Belevingsvlucht at Amsterdam airport"
)
.configure_title(
anchor="start", font="Lato", fontSize=16, fontWeight="bold"
)
.configure_view(stroke=None)
)
chart
Matplotlib representation
The airport representation can also be used in Matplotlib representations with a similar approach.
import matplotlib.pyplot as plt
from cartes.crs import Amersfoort # Official projection in the Netherlands
fig, ax = plt.subplots(
figsize=(10, 10),
subplot_kw=dict(projection=Amersfoort())
)
airports["EHAM"].plot(
ax,
footprint=True,
runways=dict(color="#f58518"), # update default parameters
labels=dict(fontsize=12),
)
ax.spines["geo"].set_visible(False)
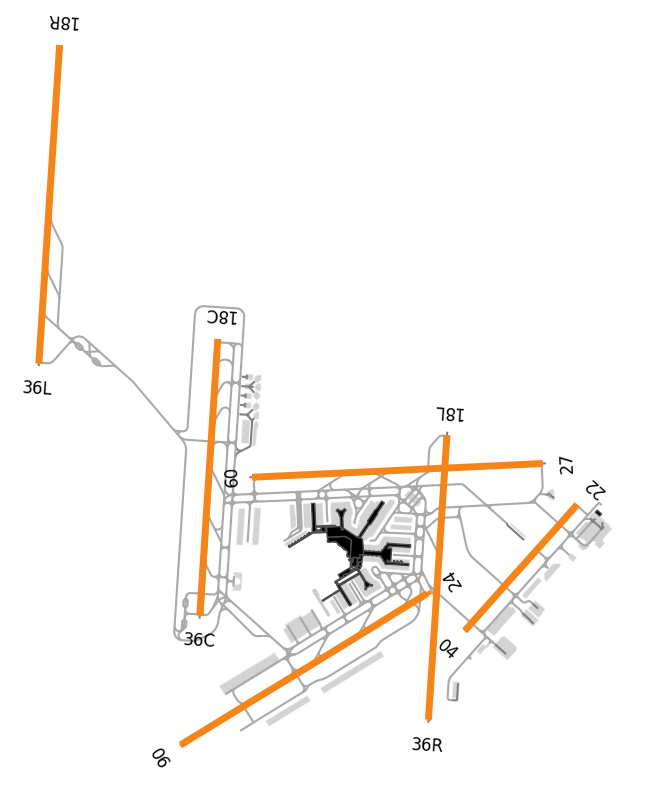
It is possible to adjust all parameters with parameters passed to the
footprint
(one entry per aeroway
tag type), runways
and labels
arguments.
fig, ax = plt.subplots(
figsize=(10, 10),
subplot_kw=dict(projection=Amersfoort())
)
airports["EHAM"].plot(
ax,
footprint=dict(taxiway=dict(color="#f58518")),
labels=dict(fontsize=12, color="crimson"),
)
ax.spines["geo"].set_visible(False)
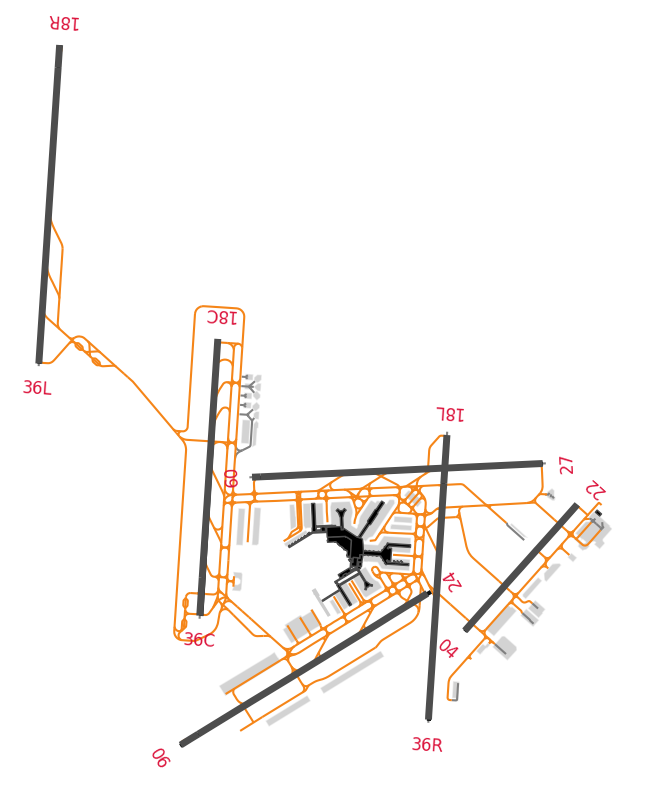